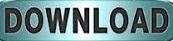

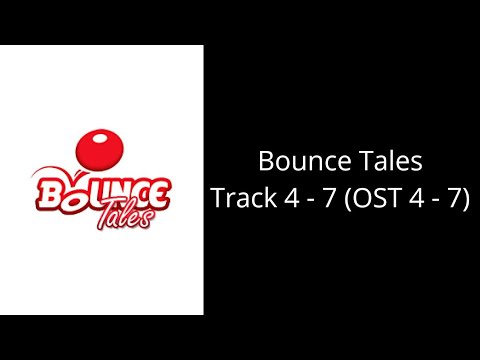
The syntax is quite unwieldy: there are 3 type arguments, and we need to specify them every time because the compiler doesn’t have enough information to infer them automatically.It only works if you have 2 parameters (of course we can adapt it for a different number of parameters, but then we need to create new methods with adequate signatures for each different arity).Well, you can guess that I wouldn’t be asking the question unless the answer was yes… The trampoline implementation demonstrated above does its job well enough, but I think it could be made more flexible and easier to use: We can now compute the factorial of large numbers, with no risk of causing a stack overflow… Admittedly, it’s not very efficient: as mentioned before, there are better ways of computing a factorial, and furthermore, computations involving BigIntegers are much slower than with ints or longs. We can then use this new function directly: Func fact = Trampoline.MakeTrampoline(Factorial) This method can’t be used directly: it returns a Bounce object, and we don’t really know what to do with this… To execute it, we use the Trampoline.MakeTrampoline method, which returns a new function on which tail recursion is applied. The recursive call to Factorial is replaced with a call to Trampoline.Recurse, which tells the trampoline that the method needs to be called again with different parameters.Instead of returning the final result directly, we call Trampoline.ReturnResult to tell the trampoline that we now have a result.Return Trampoline.Recurse(n - 1, n * product) If (n Factorial(int n, BigInteger product) Here’s a basic implementation that results directly from the definition: BigInteger Factorial(int n) Let’s see how we can transform a simple recursive algorithm, like the computation of the factorial of a number, into an algorithm that uses tail recursion (incidentally, the factorial can be computed much more efficiently with a non-recursive algorithm, but let’s assume we don’t know that…).
Bounce tails 2 java how to#
In the rest of this article, we will see how to apply this technique to a simple algorithm, using the class from Samuel Jack’s article then I’ll present another implementation of the trampoline, which I find more flexible. Samuel Jack has a good explanation of this concept on his blog. However, all is not lost! Some people had a very clever idea to work around this issue: a technique called “trampoline” (because it makes the function “bounce”) that allows to easily transform a recursive algorithm into an iterative algorithm. Unfortunately, the C# compiler doesn’t support tail recursion, which is a pity, since the CLR supports it.
Bounce tails 2 java full#
This notion being quite new to me, I won’t try to give a full course about tail recursion… much smarter people already took care of it! I suggest you follow the Wikipedia link above, which is a good starting point to understand tail recursion. So the recursion is transformed into an iteration, so it can’t cause a stack overflow. The idea is that if the recursive call is the last instruction in a recursive function, there is no need to keep the current call context on the stack, since we won’t have to go back there: we only need to replace the parameters with their new values, and jump back to the beginning of the function. Some languages, more particularly functional languages, have native support for an optimization technique called tail recursion. The trouble with the recursive approach is that it can use a lot of space on the stack: when you reach a certain recursion depth, the memory allocated for the thread stack runs out, and you get a stack overflow error that usually terminates the process ( StackOverflowException in. Regardless of the programming language you’re using, there are tasks for which the most natural implementation uses a recursive algorithm (even if it’s not always the optimal solution).
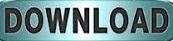